Android实现加载等待展示--进度圈
LoadingDialogUtils:
package yuduobaopromotionaledition.com.dialog;
import android.app.Activity;
import android.view.LayoutInflater;
import android.view.View;
import android.view.WindowManager;
import java.lang.ref.WeakReference;
import androidx.appcompat.app.AlertDialog;
import yuduobaopromotionaledition.com.R;
public class LoadingDialogUtils {
private static AlertDialog loadingDialog;
private static WeakReference<Activity> reference;
private static void init(Activity act) {
init(act, -1);
}
private static void init(Activity activity, int res) {
if (loadingDialog == null || reference == null || reference.get() == null || reference.get().isFinishing()) {
reference = new WeakReference<>(activity);
loadingDialog = new AlertDialog.Builder(reference.get()).create();
if (res > 0) {
View view = LayoutInflater.from(activity).inflate(res, null);
loadingDialog.setView(view);
loadingDialog.getWindow().setBackgroundDrawableResource(android.R.color.transparent);
// 去掉阴影层,这样就没有暗淡色的背景了
loadingDialog.getWindow().clearFlags(WindowManager.LayoutParams.FLAG_DIM_BEHIND);
} else {
loadingDialog.setMessage("加载中...");
}
loadingDialog.setCancelable(false);
}
}
public static void setCancelable(boolean b) {
if (loadingDialog == null) return;
loadingDialog.setCancelable(b);
}
/**
* 显示等待框
*/
public static void show(Activity act) {
show(act, false);
}
public static void show(Activity act, boolean isCancelable) {
show(act, R.layout.dialog_loading, isCancelable);
}
public static void show(Activity activity, int res, boolean isCancelable) {
init(activity, res);
loadingDialog.show();
setCancelable(isCancelable);
}
/**
* 隐藏等待框
*/
public static void dismiss() {
if (loadingDialog != null && loadingDialog.isShowing()) {
loadingDialog.dismiss();
loadingDialog = null;
reference = null;
}
}
}
dialog_loading:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent">
<ProgressBar
android:id="@+id/progress"
android:layout_width="50dp"
android:layout_height="50dp"
android:layout_centerInParent="true"
android:indeterminateDrawable="@drawable/bg_progressbar" />
</RelativeLayout>
bg_progressbar:
<?xml version="1.0" encoding="utf-8"?>
<rotate xmlns:android="http://schemas.android.com/apk/res/android"
android:drawable="@mipmap/img_loading"
android:fromDegrees="0"
android:pivotX="50%"
android:pivotY="50%"
android:toDegrees="1080" />
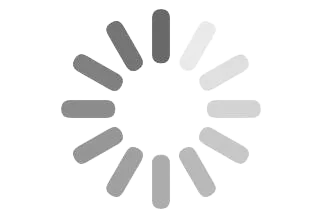
img_loading.webp
使用方法:
LoadingDialogUtils.show(requireActivity(), false);
LoadingDialogUtils.dismiss();