【PCL】(三)读写PCD文件
文章目录
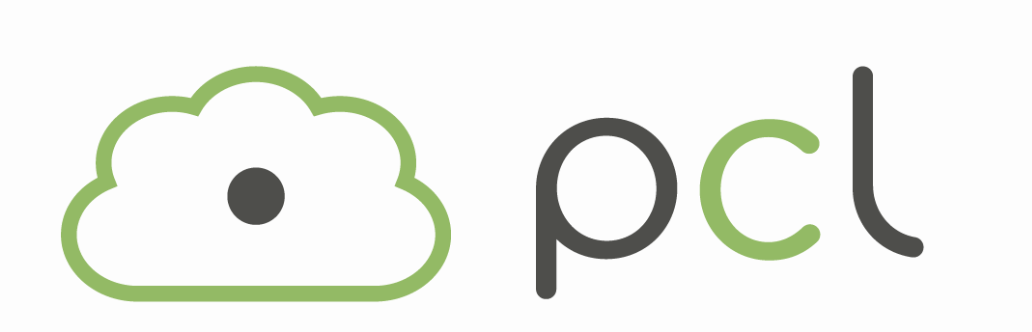
(三)读写PCD文件
写
首先,创建一个名为pcd_write.cpp
的文件,并在其中写入以下代码:
#include <iostream>
#include <pcl/io/pcd_io.h>
#include <pcl/point_types.h>
int main ()
{
pcl::PointCloud<pcl::PointXYZ> cloud;
// Fill in the cloud data
cloud.width = 5;
cloud.height = 1;
cloud.is_dense = false;
cloud.resize (cloud.width * cloud.height);
for (auto& point: cloud)
{
point.x = 1024 * rand () / (RAND_MAX + 1.0f);
point.y = 1024 * rand () / (RAND_MAX + 1.0f);
point.z = 1024 * rand () / (RAND_MAX + 1.0f);
}
pcl::io::savePCDFileASCII ("test_pcd.pcd", cloud);
std::cerr << "Saved " << cloud.size () << " data points to test_pcd.pcd." << std::endl;
for (const auto& point: cloud)
std::cerr << " " << point.x << " " << point.y << " " << point.z << std::endl;
return (0);
}
代码解释:
#include <pcl/io/pcd_io.h>
#include <pcl/point_types.h>
第一个头文件包含PCD I/O操作的定义,第二个头文件包含几个点类型结构的定义,包括我们将使用的pcl::PointXYZ
。
pcl::PointCloud<pcl::PointXYZ> cloud;
描述了我们将创建的模板化的PointCloud
结构。每个点的类型都设置为pcl::PointXYZ
,这是一个具有x
、y
和z
字段的结构。
// Fill in the cloud data
cloud.width = 5;
cloud.height = 1;
cloud.is_dense = false;
cloud.resize (cloud.width * cloud.height);
for (auto& point: cloud)
{
point.x = 1024 * rand () / (RAND_MAX + 1.0f);
point.y = 1024 * rand () / (RAND_MAX + 1.0f);
point.z = 1024 * rand () / (RAND_MAX + 1.0f);
}
使用随机值填充PointCloud
结构的XYZ,并设置适当的参数(width, height, is_dense
)。
pcl::io::savePCDFileASCII ("test_pcd.pcd", cloud);
将PointCloud
数据保存到磁盘中一个名为test_pcd.pcd
的文件中
std::cerr << "Saved " << cloud.size () << " data points to test_pcd.pcd." << std::endl;
for (const auto& point: cloud)
std::cerr << " " << point.x << " " << point.y << " " << point.z << std::endl;
用于打印出生成的数据。
将以下内容添加到CMakeLists.txt:
cmake_minimum_required(VERSION 3.5 FATAL_ERROR)
project(pcd_write)
find_package(PCL 1.2 REQUIRED)
include_directories(${PCL_INCLUDE_DIRS})
link_directories(${PCL_LIBRARY_DIRS})
add_definitions(${PCL_DEFINITIONS})
add_executable (pcd_write pcd_write.cpp)
target_link_libraries (pcd_write ${PCL_LIBRARIES})
得到可执行文件后运行它:
$ ./pcd_write
结果:
Saved 5 data points to test_pcd.pcd.
0.352222 -0.151883 -0.106395
-0.397406 -0.473106 0.292602
-0.731898 0.667105 0.441304
-0.734766 0.854581 -0.0361733
-0.4607 -0.277468 -0.916762
检查文件test_pcd.pcd的内容:
$ cat test_pcd.pcd
# .PCD v.5 - Point Cloud Data file format
FIELDS x y z
SIZE 4 4 4
TYPE F F F
WIDTH 5
HEIGHT 1
POINTS 5
DATA ascii
0.35222 -0.15188 -0.1064
-0.39741 -0.47311 0.2926
-0.7319 0.6671 0.4413
-0.73477 0.85458 -0.036173
-0.4607 -0.27747 -0.91676
扩展阅读
https://blog.csdn.net/aiyaya333/article/details/111205933
https://blog.csdn.net/gulosityer/article/details/112554056
https://blog.csdn.net/weixin_45867382/article/details/121670492
读
#include <iostream>
#include <pcl/io/pcd_io.h>
#include <pcl/point_types.h>
int main ()
{
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud (new pcl::PointCloud<pcl::PointXYZ>);
if (pcl::io::loadPCDFile<pcl::PointXYZ> ("test_pcd.pcd", *cloud) == -1) //* load the file
{
PCL_ERROR ("Couldn't read file test_pcd.pcd \n");
return (-1);
}
std::cout << "Loaded "
<< cloud->width * cloud->height
<< " data points from test_pcd.pcd with the following fields: "
<< std::endl;
for (const auto& point: *cloud)
std::cout << " " << point.x
<< " " << point.y
<< " " << point.z << std::endl;
return (0);
}
代码解释:
pcl::PointCloud<pcl::PointXYZ>::Ptr cloud (new pcl::PointCloud<pcl::PointXYZ>);
创建PointCloud boost共享指针并初始化它。
if (pcl::io::loadPCDFile<pcl::PointXYZ> ("test_pcd.pcd", *cloud) == -1) //* load the file
{
PCL_ERROR ("Couldn't read file test_pcd.pcd \n");
return (-1);
}
将PointCloud数据从磁盘加载到二进制blob中(test_pcd.pcd为上一节得到的数据)。
CMakeLists.txt
cmake_minimum_required(VERSION 3.5 FATAL_ERROR)
project(pcd_read)
find_package(PCL 1.2 REQUIRED)
include_directories(${PCL_INCLUDE_DIRS})
link_directories(${PCL_LIBRARY_DIRS})
add_definitions(${PCL_DEFINITIONS})
add_executable (pcd_read pcd_read.cpp)
target_link_libraries (pcd_read ${PCL_LIBRARIES})
得到可执行文件后运行它:
$ ./pcd_read
结果:
Loaded 5 data points from test_pcd.pcd with the following fields: x y z
0.35222 -0.15188 -0.1064
-0.39741 -0.47311 0.2926
-0.7319 0.6671 0.4413
-0.73477 0.85458 -0.036173
-0.4607 -0.27747 -0.91676
扩展阅读
https://zhuanlan.zhihu.com/p/336623565
智能指针之共享指针shared_ptr 的理解、使用
https://blog.csdn.net/aishuirenjia/article/details/91986961
参考:
https://pcl.readthedocs.io/projects/tutorials/en/master/reading_pcd.html#reading-pcd
https://pcl.readthedocs.io/projects/tutorials/en/master/writing_pcd.html#writing-pcd